1. Developing Extensions Plugins
Note: Developing plugins for SoapUI is not difficult in itself, but you do need to have a grasp of the SoapUI object model and API.
Before starting a plugin project, please take a look at the The SoapUI Object Model article and familiarize yourself with these concepts.
1.1. Sample Plugin
Note: The plugin architecture does not currently support multiple jar files. Each plugin must be deployed a single jar file to work with the plugin manager.
A sample SoapUI Search Plugin is plugin is available on GitHub.
1.2. Prerequisites
In order to develop SoapUI plugins that are compatible with the Plugin Manager, you need a 1) Java development environment, 2) Maven, and 3) A Maven archetype.
1.2.1. Development environment
For plugin development you need an environment for Java and Groovy, for example IntelliJ or Eclipse.
1.2.2. Maven
Maven is used for compiling the plugin project. See the Maven article for more information.
Note: Java Development Kit (JDK) is required for Maven.
1.2.3. Maven archetype for SoapUI plugins
As part of creating a project with Maven, you will download and use the SoapUI Plugin maven archetype.
The maven-soapui-plugin-archetype Maven archetype can be used to create both new projects, and to add to an existing project.
The archetype supports the following plugin types:
Action |
Action |
On Project level by default |
Assertion |
Custom assertion |
|
Discovery |
Custom REST Discovery method |
|
Import |
Custom Project Importer |
|
Listener |
TestRunListener |
Can be changed to any supported listener |
PanelBuilder |
PanelBuilder |
use this if you create your own TestStep |
Prefs |
Custom tab in the global Preferences |
|
RequestFilter |
Custom Request filter |
Applied to all outgoing requests |
RequestEditor |
Custom Request Editor view |
|
RequestInspector |
Custom Request Inspector tab |
|
ResponseEditor |
Custom Response Editor view |
|
ResponseInspector |
Custom Response Inspector tab |
|
SubReport |
Custom SubReport data source |
|
TestStep |
Custom TestStep |
|
ToolbarAction |
Custom Toolbar item |
|
ValueProvider |
Custom property-expansion Value Provider |
|
1.3. Creating a simple search plugin
Note: Before you start, make sure you have the necessary prerequisites installed.
1.3.1. Process
In this article we will do two main things: 1) create a basic project based on the sample plugin and try it, 2) Customize the plugin action and add features, and install the updated plugin.
- Basic Project
- Create plugin project -
mvn archetype:generate
- Build and package -
mvn install
- Install the plugin file - Add it to your ReadyAPI
- Try out the plugin - make sure it works.
- Remove the plugin again - we will replace with the customized version
- Customize the plugin
- Open the project in your favorite java development environment
- Change the
MyAction.java
class
- Edit metadata to reflect changes
- Build and package -
mvn install
- Install the plugin file - Add it to your ReadyAPI
- Try out the plugin - make sure it works
1.3.2. Create a Basic Plugin project
To create a The maven archetype will generate the basic plugin project for you;
1: Browse to the project folder (where you want to create the plugin project)
2: Open a command-prompt
3: Run the command
mvn archetype:generate -DarchetypeGroupId=com.smartbear.maven.archetypes
-DarchetypeArtifactId=soapui-plugin-archetype
-DarchetypeCatalog=http://www.eviware.com/repository/maven2
This will download and install the maven archetype and start generation of the plugin project. You will be prompted for a number of properties - set these as follows:
- groupId: "com.mycompany"
- artifactId : "my-soapui-plugin"
- version : "1.0.0"
- package : <empty >
- language : "java"
- type : "Action"
The default project is generated by maven:
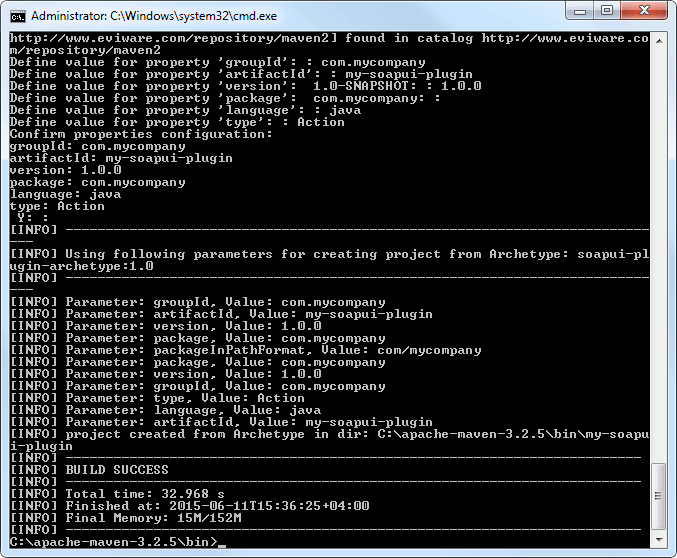
4: Open the folder my-soapui-plugin
5: Open a command-prompt
6: Run the command
mvn install
Maven builds the plugin and packages it for installation:
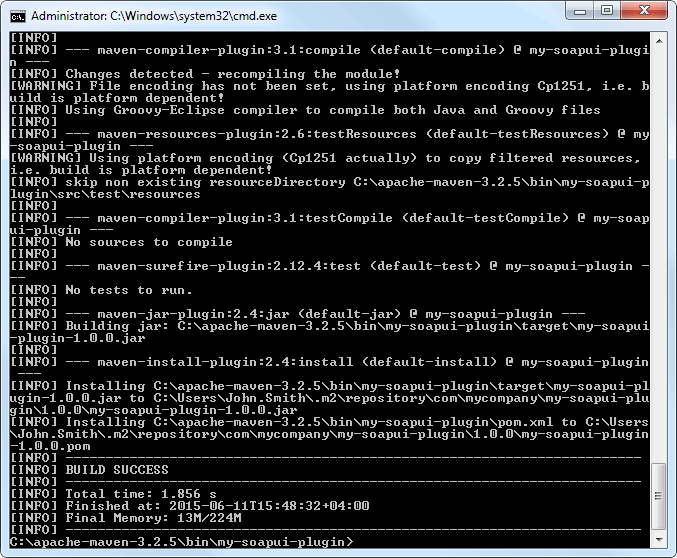
1.3.2.1. Install Plugin
Use the Plugin Manager to install the plugin file.
See the Install plugin from file section in the Plugin Manager for more information.
1.3.2.2. Try the plugin
To see the installed action:
1: Right-click on an open project in in your SoapUI workspace
The plugin action is at the bottom of the menu:
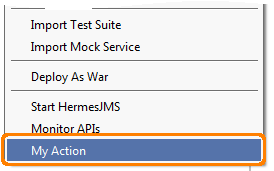
1.3.2.3. Remove Plugin
Use the Plugin Manager to uninstall the plugin file.
See the Remove plugin section in the Plugin Manager for more information.
1.3.3. Customizing the plugin
The basic sample plugin doesn't do anything useful - it only displays a a hello message. We now want to change the plugin to do a search in our project, and open a list of with the search results in the selected project.
1.3.3.1. Open project
Open the project in your java development environment.
Find the Maven generated file MyAction.java
package com.mycompany;
import com.eviware.soapui.SoapUI;
import com.eviware.soapui.impl.wsdl.WsdlProject;
import com.eviware.soapui.plugins.ActionConfiguration;
import com.eviware.soapui.support.UISupport;
import com.eviware.soapui.support.action.support.AbstractSoapUIAction;
import javax.swing.*;
@ActionConfiguration(actionGroup = ActionGroups.OPEN_PROJECT_ACTIONS)
public class MyAction extends AbstractSoapUIAction<wsdlproject> {
public MyAction() {
super("My Action", "A plugin action at the project level");
}
@Override
public void perform(WsdlProject wsdlProject, Object o) {
UISupport.showInfoMessage("Hello from [" + wsdlProject.getName() + "]!");
}
}
The default implementation of the perform
method just shows a "Hello from..." message in a popup window.
1.3.3.2. Change the action
What we'll do is change the perform
method to
- Prompt for a regular expression search string (
UISupport.prompt
)
- Do a recursive search through all items in the selected project (
findItems
)
- Match item names using the search string
- Display the results in a
ModelItemListDesktopPanel
(UISupport.showDesktopPanel
)
package com.mycompany;
import com.eviware.soapui.SoapUI;
import com.eviware.soapui.impl.wsdl.WsdlProject;
import com.eviware.soapui.model.ModelItem;
import com.eviware.soapui.plugins.ActionConfiguration;
import com.eviware.soapui.support.UISupport;
import com.eviware.soapui.support.action.support.AbstractSoapUIAction;
import com.eviware.soapui.support.components.ModelItemListDesktopPanel;
import javax.swing.*;
import java.util.ArrayList;
import java.util.List;
@ActionConfiguration(actionGroup = "EnabledWsdlProjectActions")
public class MyAction extends AbstractSoapUIAction<wsdlproject> {
public MyAction() {
super("Item Search", "A plugin for finding project items");
}
@Override
public void perform(WsdlProject wsdlProject, Object o) {
String token = UISupport.prompt( "Provide search string", "Item Search", "" );
List<modelitem> searchResult = new ArrayList<>();
findItems( searchResult, wsdlProject, token );
if( searchResult.isEmpty())
{
UISupport.showErrorMessage( "No items matching [" + token + "] found in project");
return;
}
UISupport.showDesktopPanel(new ModelItemListDesktopPanel("Search Result",
"The following items matched [" + token + "]",
searchResult.toArray(new ModelItem[searchResult.size()])));
}
private void findItems(List<modelitem> searchResult, ModelItem parent, String token)
{
for( ModelItem child : parent.getChildren())
{
if( child.getName().matches( token ))
searchResult.add( child );
findItems( searchResult, child, token );
}
}
}
We have changed some of the metadata in the constructor.
The generated PluginConfig.java
file has been updated accordingly:
package com.mycompany;
import com.eviware.soapui.plugins.PluginAdapter;
import com.eviware.soapui.plugins.PluginConfiguration;
@PluginConfiguration(groupId = "com.smartbear.soapui.plugins",
name = "Simple Search Plugin", version = "1.0.0",
autoDetect = true, description = "A simple plugin for searching for items in a project",
infoUrl = "http://olensmar.blogspot.com/2014/07/getting-started-with-new-soapui-plugin.html" )
public class PluginConfig extends PluginAdapter {
}
And also the metadata in the pom
.
<name >SoapUI Search Plugin</name >
<artifactId >soapui-search-plugin</artifactId >
<groupId >com.smartbear.soapui.plugins</groupId >
1.3.3.3. Build plugin
As before, we need to build a plugin file to install
1: Open the folder my-soapui-plugin
2: Open a command-prompt
3: Run the command
mvn install
Maven builds the plugin and packages it for installation
1.3.4. Install Plugin
See the Install plugin from file section in the Plugin Manager for more information.
1.3.5. Try out the plugin
With the plugin installed, you now have a new option ion the context menu:
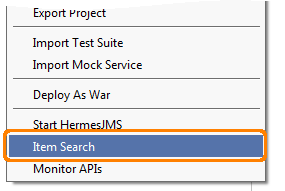
which prompts as follows when selected:
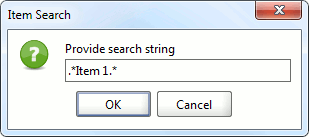
and uses the specified regular-expression to find items in the selected project and display them:
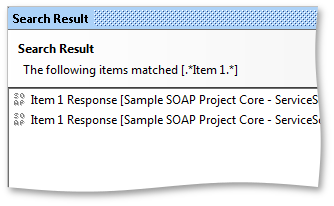
Double-clicking an item in this window opens the corresponding desktop panel for editing.
1.4. Add plugin to repository
When you are ready to publish your plugin and have it accessible from inside SoapUI, add it to the Plugin Repository. See the article Submit Plugin to Repository for more information.