If you have configured the session in HermesJMS from Getting Started, you can use SoapUI support for JMS.
First you need to use the sample project that goes with the SoapUI installation %soapui_home%/Tutorials/sample-soapui-project.xml
Open the project and right-click on interface SampleServiceSoapBinding and choose Add JMS Endpoint.
The following popup dialog will open:
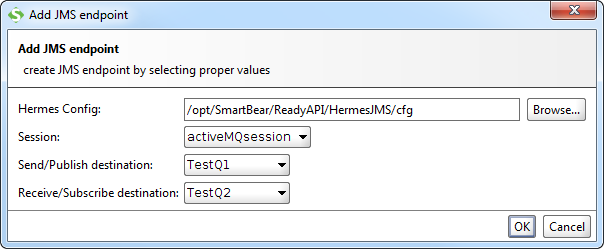
First set the Hermes Config path to the folder where hermes-config.xml is (usually .hermes), than select the above configured session and queue-topic for sending-publishing and queue-topic for receiving-subscribing. You can choose send-publish only by leaving Receive Queue field blank and also receive-subscribe only by leaving Send Queue-Topic blank.
Example:
* send only jms://activeMQsession::topic_testQ1
* receive only jms://activeMQsession::-::topic_testQ2
* send and receive jms://activeMQsession::topic_TestQ1::topic_TestQ2
Last one is useful in cases when you send something to a queue and checks topic as response immediately as it first subscribe to this topic (durable subscription ) and then send to a queue.
All combinations are allowed. Available queues and topics depend on what we have configured in HermesJMS, so if we add another queue for example it will be available in this combo box also. You can add as many JMS endpoints this way as you need.
Next we need to create a new TestSuite and TestCase and add a Soap TestRequest. Select the operation to invoke (for example the Request login), which opens the editor with a corresponding default message. Select an endpoint where the send queue and receive queues are the same and submit the request.
Special note for TIBCO EMS users: Add tibjmsadmin.jar and tibjms.jar to %SoapUI%\bin\ext folder, and restart SoapUI application after that.
Websphere MQ users:
You need the following JAR-files in SoapUI's hermes/lib folder:
- com.ibm.mq.pcf-6.1.jar
- com.ibm.mq.jar
- com.ibm.mqjms.jar
- dhbcore.jar
- connector.jar
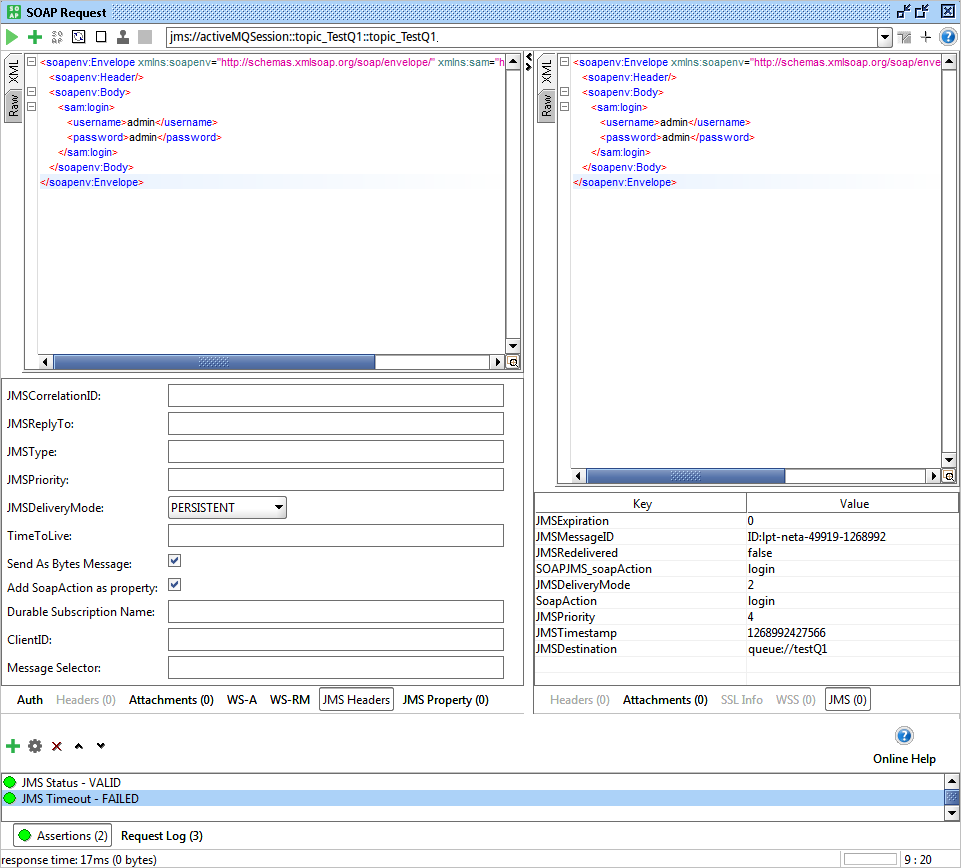
As you can see, the message we sent is shown in the response window since we sent and received from the same JMS topic (for the purpose of this example).In JMS Header inspector you can specify JMS Headers ,Time to Live (milliseconds) , to select if you want to send message as BytesMessage , to add SoapAction in property (required by some providers, Tibco EMS ...) as well as Durable Subscription Name, CliendID and you can filter response messages with Message Selector. Response has a JMS inspector showing all JMS headers and properties
You can also set custom JMS Properties for your request.
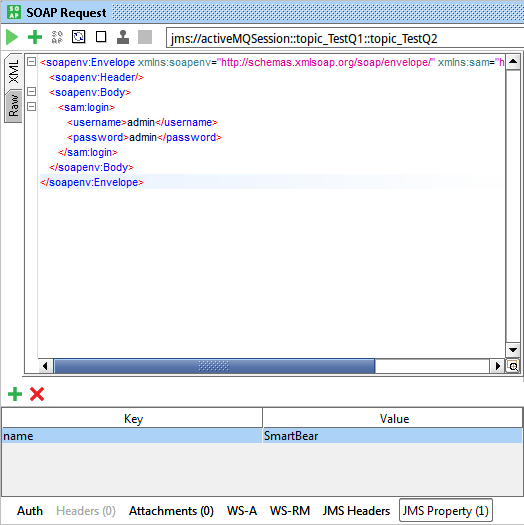
Timeout
The request Timeout property sets a limit on how long (in milliseconds) the request should wait for a response:
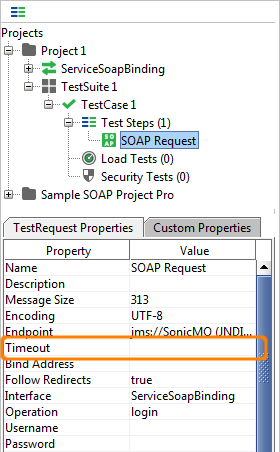
If the Timeout property is zero or empty, the request will wait indefinitely.
JMS Timeout Assertion
The JMS timeout assertion can be used to make sure that responses arrive within a particular time limit:
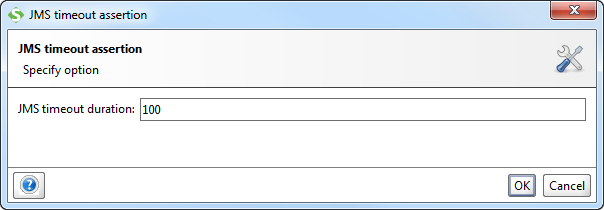
Groovy
You can also use a Groovy Script TestStep if you want something else to do with your queues and topics.
This code will use our API to browse messages from one queue without receiving it:
import com.eviware.soapui.impl.wsdl.submit.transports.jms.JMSConnectionHolder
import com.eviware.soapui.impl.wsdl.submit.transports.jms.util.HermesUtils
import com.eviware.soapui.impl.wsdl.submit.transports.jms.JMSEndpoint
import hermes.Hermes
import javax.jms.*
def jmsEndpoint = new JMSEndpoint("jms://activeMQSession::queue_testQ1::queue_testQ1");
def hermes = HermesUtils.getHermes( context.testCase.testSuite.project, jmsEndpoint.sessionName)
def jmsConnectionHolder = new JMSConnectionHolder( jmsEndpoint, hermes, false, null ,null ,null);
Session queueSession = jmsConnectionHolder.getSession();
Queue queueSend = jmsConnectionHolder.getQueue( jmsConnectionHolder.getJmsEndpoint().getSend() );
Queue queueBrowse = jmsConnectionHolder.getQueue( jmsConnectionHolder.getJmsEndpoint().getReceive() );
MessageProducer messageProducer =queueSession.createProducer( queueSend );
TextMessage textMessageSend = queueSession.createTextMessage();
textMessageSend.setText( "jms message from groovy");
messageProducer.send( textMessageSend );
textMessageSend.setText( "another jms message from groovy");
messageProducer.send( textMessageSend );
QueueBrowser qb = queueSession.createBrowser(queueBrowse);
Enumeration en= qb.getEnumeration();
while(en.hasMoreElements()){
TextMessage tm = (TextMessage)en.nextElement();
log.info tm.getText()
}
jmsConnectionHolder.closeAll()// don't forget to close session and connection