NOTE: This page contains information on standalone SoapUI Pro that has been replaced with ReadyAPI.
To try enhanced scripting functionality, feel free to download a ReadyAPI trial from our website.
SoapUI provides extensive options for scripting, using either Groovy or Javascript (since SoapUI 3.0) as its scripting language, which to use is set a the project level in the project details tab at the bottom left. The majority of the documentation available here will be for the Groovy language, as it greatly simplifies the scripting of Java APIs (you can get more information, tutorials, etc on the Groovy Web Site). An overview of how to use JavaScript instead is further down in this document.
Scripts can be used at the following places in SoapUI:
- As part of a TestCase with the Groovy Script TestStep, allowing your tests to perform virtually any desired functionality
- Before and after running a TestCase or TestSuite for initializing and cleaning up before or after running your tests.
- When starting/stopping a MockService to initializing or cleaning-up MockService state
- When opening/closing a Project, for initializing or cleaning-up Project related settings
- As a dynamic DataSource or DataSink with the corresponding DataSource/DataSink test steps
- For providing dynamic MockOperation dispatching.
- For creating dynamic MockResponse content
- For creating arbitrary assertions with the Script Assertion
- To extend SoapUI itself (see Extending SoapUI), for adding arbitrary functionality to the SoapUI core
All scripts have access to a number of situation-specific variables, always including a log object for logging to the Groovy Log and a context object for perform context-specific PropertyExpansions or property handling if applicable. A context-specific variable is always available for directly accessing the SoapUI object model.
1. Script Editors
Script editors are generally available as inspectors at the bottom of the corresponding objects editor, each having a run button, a drop-down edit menu (same as the right-click popup), an information label, and a help button;
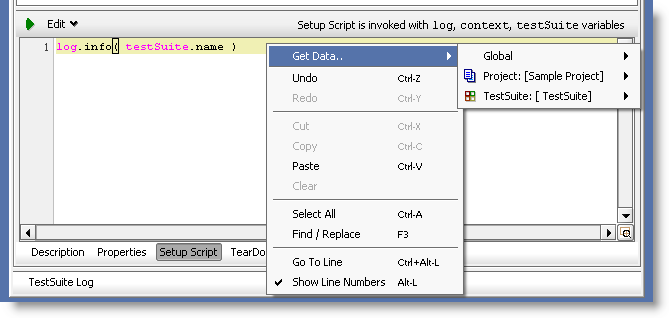
The popup-menu (as shown above) contains standard edit-related actions and will in ReadyAPI contain a "Get Data" menu option that expands to show all properties available within the current scope. Selecting a property (or the option to create a new one) will eventually create a script to get the variable, for example
def test = context.expand( '${#Project#test}' )
Which gets the Project-level "test" property.
It is also possible to drag a property from the navigator tree when it is in Property Mode into the script; if the dragged property is "within scope" (i.e. can be accessed via property expansion), the corresponding access script will be created at the caret location
2. Groovy Script Library
You can have a central library of Groovy Classes that can be accessed from any script, which can be useful for centralizing common tasks and functionality and for creating extensions.
Groovy Script Library
The Script Library can be used as follows;
- Specify which directory to use in the SoapUI Preferences tab (default is /scripts). SoapUI will check this directory for files with the "Groovy" extension and compile these upon startup. The directory is then checked periodically (every 5 seconds) for updates and new or existing scripts are compiled and re-compiled if necessary.
- Scripts should be placed in directories named after their containing package, that is a script in the package soapui.demo should be in a soapui/demo directory under the specified scripts directory.
- The compiled classes are added to the parent class loader of all Groovy scripts, you can access them as standard java classes.
Remember that the script files must be valid classes, not just arbitrary scripts. So as an example, lets setup one of these Groovy objects. First, create a directory (e.g. C:\GroovyLib
). Then add a Callee.groovy
file in that directory with the following content:
package readyapi.demo
//Callee.groovy
class Callee {
String hello() {
return "Hello world! "
}
def static salute( who, log ) { log.info "Hello again $who!" }
}
Now let's setup SoapUI to load up your Groovy library. Set File > Preferences > SoapUI tab > Script Library.
So we would set that to "C:\GroovyLib" in our example.
Then we restart SoapUI to pick up the library script.
Now if we create a Groovy Script Step in a TestCase, we can use the above class from the library with the following:
//Caller.groovy
c = new Callee()
log.info c.hello()
Running this from within the Groovy Editor will show the following in the Groovy Editors log:
Tue MONTH 29 10:56:08 EST YEAR:INFO:Hello world!
Modifying Script File
If we modify the Callee.groovy
file:
package readyapi.demo
//Callee.groovy
class Callee {
String hello() {
return "Hello world! "
}
String hello(String who) {
return "Hello $who"
}
def static salute( who, log ) { log.info "Hello again $who!" }
}
SoapUI will pick up the modified file (once it has been saved), which is seen in the log:
Tue MONTH 29 10:56:08 EST YEAR:INFO:C:\GroovyLib\Callee.groovy is new or has changed, reloading...
We also change the script:
//Caller.groovy
c = new Callee()
log.info c.hello("Mike")
And we get:
Tue MONTH 29 10:56:08 EST YEAR:INFO:Hello, Mike!
Static Method
We can also call the static salute method:
readyapi.demo.Callee.salute( "Mike", log )
Which will produce the following output:
Tue MONTH 29 10:56:08 EST YEAR:INFO:Hello again Mike!
3. Code Templates
ReadyAPI 2.5 adds rudimentary support for configurable code templates which will be activated when typing the correspondig template ID and pressing Ctrl-Space in an editor. Templates can be added/changed in the Preferences\Code Templates page, the following templates are available by default:
- grut - def groovyUtils = new com.eviware.soapui.support.GroovyUtils( context )\n|
- xhre - def holder = groovyUtils.getXmlHolder( "|#Response" )
The '|' designates the position of the caret after inserting the template.
4. JavaScript support
SoapUI 3.0 adds support for JavaScript as an alternative scripting language. When configured, all scripts in the containing project will be interpreted using a JavaScript engine (Rhino) instead (read more about the Rhino engine and supported features at ... ). For those of you used to JavaScript this might be an easier language to get started with.
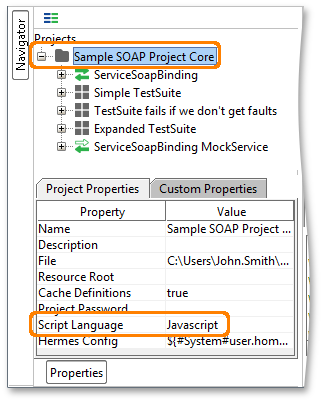
A note on the choice of Groovy:
When we started to provide scripting possibilities in 2006, Groovy was the natural language of choice. Now this has changed and there are many viable alternatives to Groovy scripting API (JRuby, Jython, Javascript, etc), but since we have made our own optimizations for the Groovy ClassLoaders which would not be available for these other languages, we have opted to stick to Groovy instead of providing "sub-optimal" support for other languages.